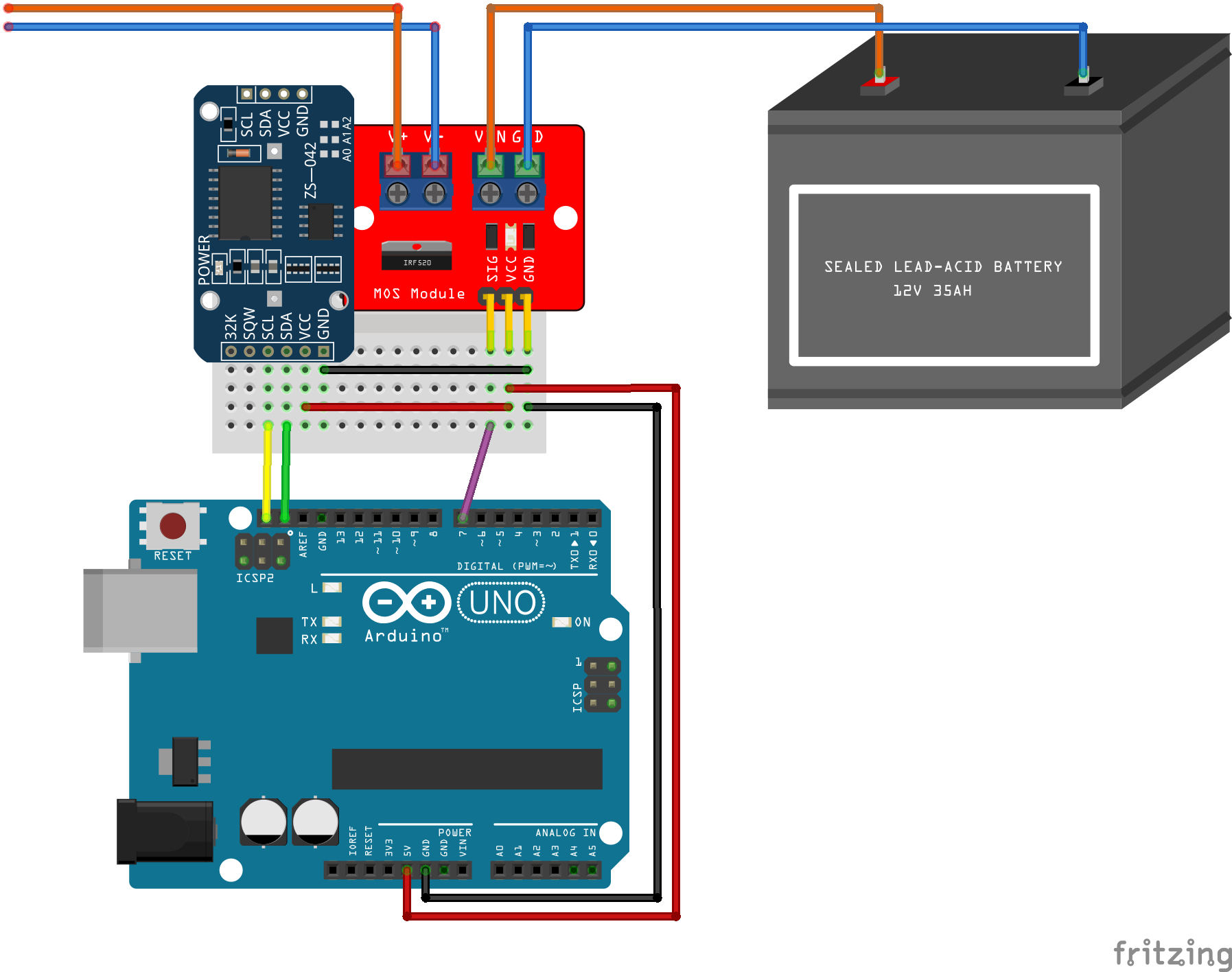
#define DEBUG false
#include "RTClib.h"
RTC_DS3231 rtc;
#define SWITCHCMD 7
#define ON true
#define OFF false
char daysOfTheWeek[7][12] = {"Lundi", "Mardi", "Mercredi", "Jeudi", "Vendredi", "Samedi", "Dimanche"};
int daysOff[7] = {5, 6};
struct prog {
int Heure;
int Minute;
boolean Etat;
};
struct prog Calendar [] = {
{ 0, 0, OFF },
{ 7, 45, ON },
{ 8, 0, OFF },
{ 9, 45, ON },
{ 10, 0, OFF },
{ 11, 45, ON },
{ 13, 25, OFF },
{ 15, 15, ON },
{ 15, 30, OFF },
{ 17, 20, ON },
{ 17, 45, OFF }
};
boolean _state = false;
const int Day = 1;
const int Month = 2;
const int Year = 3;
const int Hour = 4;
const int Minute = 5;
void setup () {
Serial.begin(115200);
while (!Serial);
pinMode(SWITCHCMD, OUTPUT);
if (! rtc.begin()) {
Serial.println("!!! STOP !!! Couldn't find RTC");
while (1);
} else {
Serial.println("- RTC initialized");
}
if (rtc.lostPower()) {
Serial.println("RTC lost power, let's set the time!");
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// rtc.adjust(DateTime(2014, 1, 21, 3, 0, 0));
}
Serial.println("- End of Setup");
}
void loop () {
digitalWrite(SWITCHCMD, checkState());
Serial.print(".");
delay(15000);
}
boolean checkState() {
int CalendarSize = sizeof(Calendar)/sizeof(*Calendar);
int daysOffSize = sizeof(daysOff)/sizeof(*daysOff);
DateTime now = rtc.now();
if(DEBUG) {
Serial.print("*** Time = ");
Serial.print(now.hour());
Serial.print(" H ");
Serial.print(now.minute());
Serial.print(" min");
Serial.println(" ***");
Serial.print("*** Day = ");
Serial.print(daysOfTheWeek[now.dayOfTheWeek()-1]);
Serial.print(" ");
Serial.print(now.day());
Serial.print("/");
Serial.print(now.month());
Serial.print("/");
Serial.print(now.year());
Serial.println(" ***");
}
// Test from calandar
for (int i=0; i<CalendarSize; i++) {
double nowSec = now.hour()*3600+now.minute()*60+now.second();
double checSec = Calendar[i].Heure*3600+Calendar[i].Minute*60;
if(DEBUG) {
Serial.print("Check !!! Now = "); Serial.print(nowSec); Serial.print(" check = "); Serial.println(checSec);
}
if (nowSec > checSec) {
_state = Calendar[i].Etat;
if(DEBUG) {
Serial.print("Bingo !!!"); Serial.print(Calendar[i].Heure); Serial.print(":"); Serial.print(Calendar[i].Minute); Serial.print(" "); Serial.println(Calendar[i].Etat);
}
}
}
// Test for dayOff
for (int j=0; j<daysOffSize; j++) {
if(now.dayOfTheWeek() == daysOff[j] ) {
_state = false;
if(DEBUG) {
Serial.print(" *** This is dayOff !!!");
}
}
}
return _state;
}